Coding in VB.net
Page 1 of 1
Coding in VB.net
Credits are appreciated, but are not mandatory
Hey there, due multiply request I decided to make this tutorial for making a trainer in VB
In this tutorial I will keep it to the basic (No complicated ASM hooks) and will explain how to setup your own trainer
Requirements:
Microsoft Visual Basic 2010 Express (2010 recommended)
Step 1 : Creating a project
Go File>New Project (or press ctrl+n) and select the "Windows Forms Application"
Put in a name you like or preffer (or just leave it "WindowsApplication1") and press OK
After you press OK the program will start loading, when its finished it will look like this
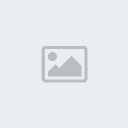
(if you do not c the ToolBox window, press Ctrl+alt+x or go View>Other windows>toolbox)
You now created your own un-finished trainer, wich actually can be run already (F5 = testing/debugging)
Step 2 : Adding the necessary things
First of all its suggested to download: http://localhostr.com/file/2MO0e6A/WINAPI.vb file
And then add it by doing Project>Add existing item (or ctrl+d), and then select the WINAPI.vb you just downloaded
If you did it correctly you will see that the file is added like this
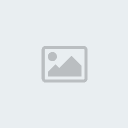
Also, you can ignore the 12 Errors it gives, we will fix that later on
Step 3 : Hooking Maple
Now we need to set up a few things so we can use hacks in Maplestory
First off all we need to add a piece of code, you can reach the code page by double clicking on your form (@Form1.vb)
Something like this will appear:
Now look in your toolbox for a "Timer", if you found it, then drag and drop it on your form
Under your form Timer1 will now apear, when its done loading, double click the Timer1
You will see a new piece of code has been added looking like this
Now we still need to start the timer, we want the timer to start when the Form1 opens
So after "Private Sub Form1_Load", we will add this
If you did everything correctly, your code will now look like this
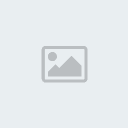
Step 4 : Hacks! Finnaly
Now we will start adding hacks, the simplest way of adding hacks would be using a checkbox, you can add them the same way as an Timer
Although there will be a small difference now, you can use you mouse to drag the checkbox to the position you preffer
After you added a checkbox, double click so a new piece of code will be generated called "Private Sub CheckBox1_CheckedChanged"
In this Sub we will add Super Tubi
The super tubi script looks like this
So in VB we will do exactly the same
First we need to define the address, we do that with this piece of code
Now we need to know if we need to enable, or disable the hack, dependig on the checkbox, wich is actually quite simple
To figure out what we need to do to enable the hack, we will look back at the CE script, and see that we need to write 5 bytes all with the value 90 (In hexadecimal)
First we will define that, we use this way to do that
We use the following function for that
The only thing thats left to do is adding the disable part, the only difference of the disable part are the bytes, instead of 5 times 90, we now need these bytes
If you putted everything together like it should, it should now look like this
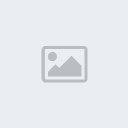
Final Step: Saving and Building
Now we need to save our Trainer, we can do this by pressing Ctrl+shift+z (or File>Save All)
You will be asked were you want to save your trainer, Remember this! you will need it later on
After you saved it, you can go Debug>Build [Your project name here], this will make an ready to use .exe
It will get saved on the location you picked \bin\Release, try to find that map
After you found that map there will be a file called "WindowsApplication1.exe", this is your trainer ! :3
Its ready to use, you can now test it on MapleStory
(Dont forget to inject a bypass though)
I hoped this helped you guys out
Admin
Expect more to come!
Hey there, due multiply request I decided to make this tutorial for making a trainer in VB
In this tutorial I will keep it to the basic (No complicated ASM hooks) and will explain how to setup your own trainer
Requirements:
Microsoft Visual Basic 2010 Express (2010 recommended)
- Code:
http://www.microsoft.com/express/Downloads/#2010-Visual-Basic
Step 1 : Creating a project
Go File>New Project (or press ctrl+n) and select the "Windows Forms Application"
Put in a name you like or preffer (or just leave it "WindowsApplication1") and press OK
After you press OK the program will start loading, when its finished it will look like this
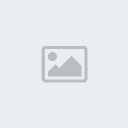
(if you do not c the ToolBox window, press Ctrl+alt+x or go View>Other windows>toolbox)
You now created your own un-finished trainer, wich actually can be run already (F5 = testing/debugging)
Step 2 : Adding the necessary things
First of all its suggested to download: http://localhostr.com/file/2MO0e6A/WINAPI.vb file
And then add it by doing Project>Add existing item (or ctrl+d), and then select the WINAPI.vb you just downloaded
If you did it correctly you will see that the file is added like this
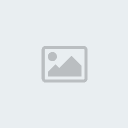
Also, you can ignore the 12 Errors it gives, we will fix that later on
Step 3 : Hooking Maple
Now we need to set up a few things so we can use hacks in Maplestory
First off all we need to add a piece of code, you can reach the code page by double clicking on your form (@Form1.vb)
Something like this will appear:
- Code:
Public Class Form1
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
End Sub
End Class
- Code:
Public Shared ProcessHandle As IntPtr = 0
Public Shared hWnd As IntPtr = 0
Public Shared ProcessID As UInt32 = 0
Public Shared MapleStory As Process
Now look in your toolbox for a "Timer", if you found it, then drag and drop it on your form
Under your form Timer1 will now apear, when its done loading, double click the Timer1
You will see a new piece of code has been added looking like this
- Code:
Private Sub Timer1_Tick(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Timer1.Tick
End Sub
- Code:
Try
Dim ProcessList As Process() = Process.GetProcesses
Dim i As Integer = 0
While i < ProcessList.Length
If ProcessList(i).ProcessName.ToString = "MapleStory" Then
MapleStory = ProcessList(i)
End If
i = i + 1
End While
hWnd = MapleStory.MainWindowHandle
ProcessID = MapleStory.Id
ProcessHandle = WINAPI.OpenProcess(&H38, 1, ProcessID)
Catch ex As Exception
End Try
Now we still need to start the timer, we want the timer to start when the Form1 opens
So after "Private Sub Form1_Load", we will add this
- Code:
Timer1.Interval = 1000
Timer1.Start()
If you did everything correctly, your code will now look like this
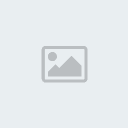
Step 4 : Hacks! Finnaly

Now we will start adding hacks, the simplest way of adding hacks would be using a checkbox, you can add them the same way as an Timer
Although there will be a small difference now, you can use you mouse to drag the checkbox to the position you preffer
After you added a checkbox, double click so a new piece of code will be generated called "Private Sub CheckBox1_CheckedChanged"
In this Sub we will add Super Tubi
The super tubi script looks like this
- Code:
[Enable]
00A6606F:
db 90 90 90 90 90
[Disable]
00A6606F:
db 89 BE B8 20 00 00
So in VB we will do exactly the same
First we need to define the address, we do that with this piece of code
- Code:
Dim Addy As IntPtr = &HA6606F
Now we need to know if we need to enable, or disable the hack, dependig on the checkbox, wich is actually quite simple
- Code:
If CheckBox1.Checked Then
Else
End If
To figure out what we need to do to enable the hack, we will look back at the CE script, and see that we need to write 5 bytes all with the value 90 (In hexadecimal)
First we will define that, we use this way to do that
- Code:
Dim buffer As Byte() = New Byte() {&H90, &H90, &H90, &H90, &H90}
We use the following function for that
- Code:
WINAPI.WriteProcessMemory(Addy, buffer, buffer.Length)
The only thing thats left to do is adding the disable part, the only difference of the disable part are the bytes, instead of 5 times 90, we now need these bytes
- Code:
{&H89, &HBE, &HB8, &H20, &H0, &H0}
If you putted everything together like it should, it should now look like this
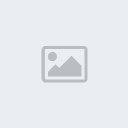
Final Step: Saving and Building
Now we need to save our Trainer, we can do this by pressing Ctrl+shift+z (or File>Save All)
You will be asked were you want to save your trainer, Remember this! you will need it later on
After you saved it, you can go Debug>Build [Your project name here], this will make an ready to use .exe
It will get saved on the location you picked \bin\Release, try to find that map
After you found that map there will be a file called "WindowsApplication1.exe", this is your trainer ! :3
Its ready to use, you can now test it on MapleStory

I hoped this helped you guys out
Admin
Expect more to come!

Page 1 of 1
Permissions in this forum:
You cannot reply to topics in this forum